Creating Email Verification in PHP
April 30, 2016
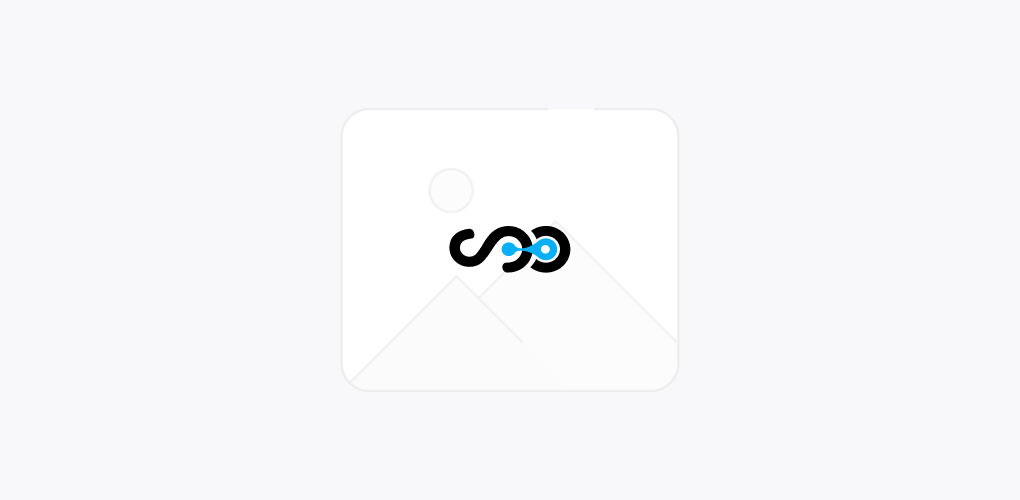
PHP is used for developing web applications; we all are aware about this. However, PHP can be used for other things as well. Today, in this blog post; we will see how emails can be verified using PHP.
The common security feature during user registration process is email verification. It is necessary to create it as per the industry best practices in order to avoid certain security risks.
Here, we will see how email verification can be created with PHP?

First of all, let’s begin with registration form:
<form method=”post” action=”http://mydomain.com/registration/”>
<fieldset class=”form-group”>
<label for=”fname”>First Name:</label>
<input type=”text” name=”fname” class=”form-control” required />
</fieldset>
<fieldset class=”form-group”>
<label for=”lname”>Last Name:</label>
<input type=”text” name=”lname” class=”form-control” required />
</fieldset>
<fieldset class=”form-group”>
<label for=”email”>Last name:</label>
<input type=”email” name=”email” class=”form-control” required />
</fieldset>
<fieldset class=”form-group”>
<label for=”password”>Password:</label>
<input type=”password” name=”password” class=”form-control” required />
</fieldset>
<fieldset class=”form-group”>
<label for=”cpassword”>Confirm Password:</label>
<input type=”password” name=”cpassword” class=”form-control” required />
</fieldset>
<fieldset>
<button type=”submit” class=”btn”>Register</button>
</fieldset>
</form>
Later on, we will use this database structure:
CREATE TABLE IF NOT EXISTS `user` (
`id` INT(10) NOT NULL AUTO_INCREMENT PRIMARY KEY,
`fname` VARCHAR(255) ,
`lname` VARCHAR(255) ,
`email` VARCHAR(50) ,
`password` VARCHAR(50) ,
`is_active` INT(1) DEFAULT ‘0’,
`verify_token` VARCHAR(255) ,
`created_at` TIMESTAMP,
`updated_at` TIMESTAMP,
);
Once this form is submitted then the input is to be checked. After input verification, new user is to be created:
// Validation rules
$rules = array(
‘fname’ => ‘required|max:255’,
‘lname’ => ‘required|max:255′,
’email’ => ‘required’,
‘password’ => ‘required|min:6|max:20’,
‘cpassword’ => ‘same:password’
);
$validator = Validator::make(Input::all(), $rules);
// If input not valid, go back to registration page
if($validator->fails()) {
return Redirect::to(‘registration’)->with(‘error’, $validator->messages()->first())->withInput();
}
$user = new User();
$user->fname = Input::get(‘fname’);
$user->lname = Input::get(‘lname’);
$user->password = Input::get(‘password’);
// You will generate the verification code here and save it to the database
// Save user to the database
if(!$user->save()) {
// If unable to write to database for any reason, show the error
return Redirect::to(‘registration’)->with(‘error’, ‘Unable to write to database at this time. Please try again later.’)->withInput();
}
// User is created and saved to database
// Verification e-mail will be sent here
// Go back to registration page and show the success message
return Redirect::to(‘registration’)->with(‘success’, ‘You have successfully created an account. The verification link has been sent to e-mail address you have provided. Please click on that link to activate your account.’);
Once the registration is complete, the account will remain inactive till the email has been verified. This feature confirms that user is the real owner of the email id and this helps to prevent spam, unknown email usage as well as information leaks.
It is a very simple process, once the new user is created; an email containing verification link is to be sent to the email id that was entered during registration process. Users will be unable to login and use web applications till the time he/she clicks on verification link and confirms the email address.
Regarding verification links, there are few thinks to note down: It must have a randomly generated token which must be long enough and useful for certain time period. The user identified must also be included so that potential attacks could not brute force across multiple accounts.
Wind up:
Whatever codes we have included here are only for educational purpose and are not completely tested. So, if you wish to use them in any web application then kindly test the same.
These are written in Laravel Framework however can be easily used in PHP. The verification code is valid up to 48 hours hence it will be good to use such application that can eliminate inactive users with expired verification codes.
Hope you liked this post. For more such updates about PHP; stay in touch with Softqube Technologies; provider of cost effective php development services.
Share on