Kubernetes vs. the World: Container Orchestration Faceoff
September 5, 2023
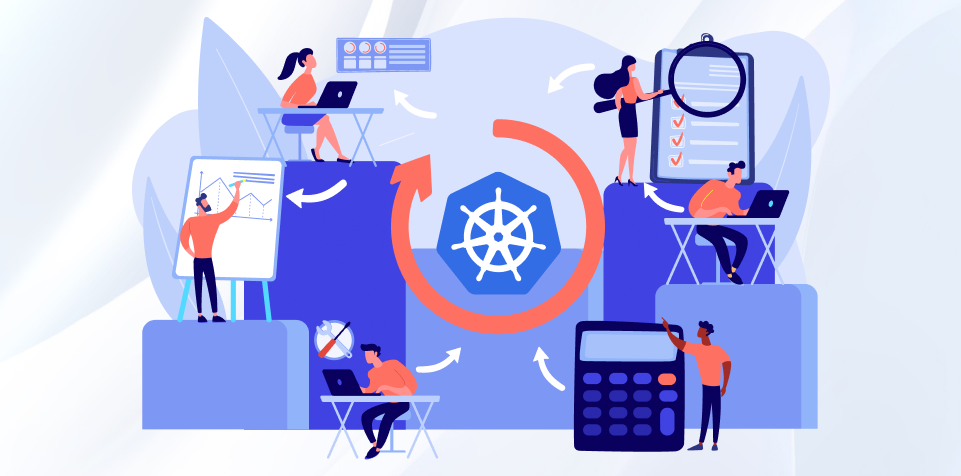
What is Kubernetes?
Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications. It was originally developed by Google and is now maintained by the Cloud Native Computing Foundation (CNCF). Kubernetes provides a platform-agnostic solution to manage containerized workloads and services.
Why Use Kubernetes?
Kubernetes offers several key benefits for containerized application management:
1. Automated Orchestration: Kubernetes automates the deployment and scaling of applications, making it easier to manage and maintain containerized workloads.
2. High Availability: Kubernetes ensures high availability by automatically distributing workloads across multiple nodes and rescheduling containers if a node fails.
3. Horizontal Scaling: Kubernetes can dynamically scale applications based on load, ensuring optimal resource utilization.
4. Self-Healing: Kubernetes automatically restarts failed containers and replaces them to maintain the desired state.
5. Declarative Configuration: Define the desired state of your application using YAML manifests, and Kubernetes will handle the actual state.
Use Cases of Kubernetes
Kubernetes is versatile and suitable for various use cases, including:
1. Application Deployment and Management
Kubernetes simplifies deploying and managing applications, enabling teams to focus on building features rather than dealing with infrastructure complexities.
2. Microservices Architecture
Kubernetes supports microservices-based applications, allowing independent deployment, scaling, and versioning of different microservices.
3. Continuous Deployment
Kubernetes integrates with continuous integration and continuous deployment (CI/CD) tools, streamlining the process of delivering updates to applications.
4. Hybrid and Multi-Cloud Environments
bernetes provides a consistent platform to run applications across different cloud providers and on-premises environments.
How Kubernetes Works
Kubernetes uses a master-worker node architecture:
Master Node
The master node controls the Kubernetes cluster and makes global decisions about the cluster’s state. Key components on the master node include:
- API Server: Exposes the Kubernetes API and acts as the front-end for the control plane.
- Controller Manager: Runs various controllers that handle routine tasks such as node and endpoint monitoring.
- Scheduler: Assigns pods to nodes based on resource requirements and other constraints.
- etcd: A distributed key-value store that stores the cluster’s configuration data.
Worker Nodes
Worker nodes (also called minion nodes) are where containers are scheduled and run. Each worker node communicates with the master node. Key components on worker nodes include:
- Kubelet: Communicates with the API server and manages containers on the node.
- Container Runtime: The software responsible for running containers, such as Docker or containerd.
- kube-proxy: Manages network connectivity between services within the cluster.
Kubernetes Cluster Diagram
Here’s a simplified diagram of a Kubernetes cluster:
Kubernetes Fundamental Concepts
1. Pods
Pods are the smallest deployable units in Kubernetes, representing one or more containers that share storage and network resources. Pods are often co-located on the same node and communicate with each other via localhost.
2. ReplicaSets
ReplicaSets ensure that a specified number of identical pods are running at all times, even in the face of failures. They are used to maintain the desired number of replicas for a specific pod template.
3. Deployments
Deployments provide declarative updates to ReplicaSets, managing the process of creating and updating pods. They are the recommended way to manage the lifecycle of pods.
4. Services
Services enable network access to pods, allowing them to communicate with each other and external clients. Services abstract the underlying pod IP addresses and provide a stable endpoint.
5. ConfigMaps and Secrets
ConfigMaps and Secrets store configuration data and sensitive information, respectively, which can be injected into containers. They allow for decoupling configuration from the container image.
Kubernetes Deployment
Deploying Node.js Project on Minikube Cluster
This documentation outlines the steps to deploy MongoDB and Mongo Express on Kubernetes using the provided YAML configuration files.
Prerequisites
Before deploying the project, make sure you have the following prerequisites:
1. Kubernetes cluster is set up and running.
2. kubectl is installed and configured to access the Kubernetes cluster.
Step 1: Set Up Kubernetes Cluster
To set up the Kubernetes cluster, follow the steps below:
1. Install a Container Runtime (e.g., Docker)
Ensure that you have a container runtime installed on your system. Docker is a commonly used container runtime.
2. Install a Kubernetes Management Tool (e.g., Minikube, k3s, kubeadm)
Choose a Kubernetes management tool suitable for your environment and follow the installation instructions.
Example: Installing Minikube (For Local Development)
For local development, you can use Minikube. Install Minikube using the following command:
# Install Minikube (Linux)
curl -LO https://storage.googleapis.com/minikube/releases/latest/minikube-linuxamd64 \
&& sudo install minikube-linux-amd64 /usr/local/bin/minikube
# Install Minikube (macOS)
brew install minikube
3. Start the Kubernetes Cluster (Minikube)
After installing Minikube, start the Kubernetes cluster using the following command:
minikube start
Step 2: Install and Configure kubectl
To install and configure kubectl, follow the steps below:
1. Install kubectl
Install kubectl using your package manager or download the binary from the official Kubernetes release:
# Install `kubectl` (Linux)
sudo apt-get update && sudo apt-get install -y kubectl
# Install `kubectl` (macOS)
brew install kubectl
2. Configure kubectl to Access the Kubernetes Cluster
Configure kubectl to access the Kubernetes cluster created in Step 1 (e.g., Minikube):
# Set `kubectl` context to Minikube
kubectl config use-context minikube
Step 3: Deploy MongoDB
1. Create the Secret for MongoDB Root Credentials
# (Content of mongo-secret.yaml)
apiVersion: v1
kind: Secret
metadata:
name: mongodb-secret
type: Opaque
data:
mongo-root-username: dXNlcm5hbWU=
mongo-root-password: cGFzc3dvcmQ=
Apply the MongoDB Secret using the following command:
kubectl apply -f mongo-secret.yaml
2. Deploy MongoDB Deployment and Service
# (Content of mongo.yaml)
apiVersion: apps/v1
kind: Deployment
metadata:
name: mongodb-deployment
labels:
app: mongodb
spec:
replicas: 1
selector:
matchLabels:
app: mongodb
template:
metadata:
labels:
app: mongodb
spec:
containers:
- name: mongodb
image: mongo
ports:
- containerPort: 27017
env:
- name: MONGO_INITDB_ROOT_USERNAME
valueFrom:
secretKeyRef:
name: mongodb-secret
key: mongo-root-username
- name: MONGO_INITDB_ROOT_PASSWORD
valueFrom:
secretKeyRef:
name: mongodb-secret
key: mongo-root-password
---
apiVersion: v1
kind: Service
metadata:
name: mongodb-service
spec:
selector:
app: mongodb
ports:
- protocol: TCP
port: 27017
targetPort: 27017
Apply the MongoDB Deployment and Service using the following command:
kubectl apply -f mongo.yaml
Step 4: Deploy Mongo Express
1. Deploy Mongo Express Deployment and Service
# (Content of mongo-express.yaml)
apiVersion: apps/v1
kind: Deployment
metadata:
name: mongo-express
labels:
app: mongo-express
spec:
replicas: 1
selector:
matchLabels:
app: mongo-express
template:
metadata:
labels:
app: mongo-express
spec:
containers:
- name: mongo-express
image: mongo-express
ports:
- containerPort: 8081
env:
- name: ME_CONFIG_MONGODB_ADMINUSERNAME
valueFrom:
secretKeyRef:
name: mongodb-secret
key: mongo-root-username
- name: ME_CONFIG_MONGODB_ADMINPASSWORD
valueFrom:
secretKeyRef:
name: mongodb-secret
key: mongo-root-password
- name: ME_CONFIG_MONGODB_SERVER
valueFrom:
configMapKeyRef:
name: mongodb-configmap
key: database_url
---
apiVersion: v1
kind: Service
metadata:
name: mongo-express-service
spec:
selector:
app: mongo-express
type: LoadBalancer
ports:
- protocol: TCP
port: 8081
targetPort: 8081
nodePort: 30000
Apply the Mongo Express Deployment and Service using the following command:
kubectl apply -f mongo-express.yaml
Step 5: Configure the Database URL ConfigMap
# (Content of mongo-config.yaml)
apiVersion: v1
kind: ConfigMap
metadata:
name: mongodb-configmap
data:
database_url: mongodb-service
Apply the Database URL ConfigMap using the following command:
kubectl apply -f mongo-config.yaml
Cleanup
To clean up the deployed services, use the following commands:
kubectl delete -f mongo-express.yaml
kubectl delete -f mongo.yaml
kubectl delete -f mongo-secret.yaml
kubectl delete -f mongo-config.yaml
Conclusion
Kubernetes is a powerful container orchestration platform that simplifies the management of containerized applications. It provides scalability, high availability, and automation, making it a popular choice for modern cloud-native applications.
For more detailed information, refer to the official Kubernetes documentation: https://kubernetes.io/docs/