How to Read CSV Data in PHP Array?
April 1, 2016
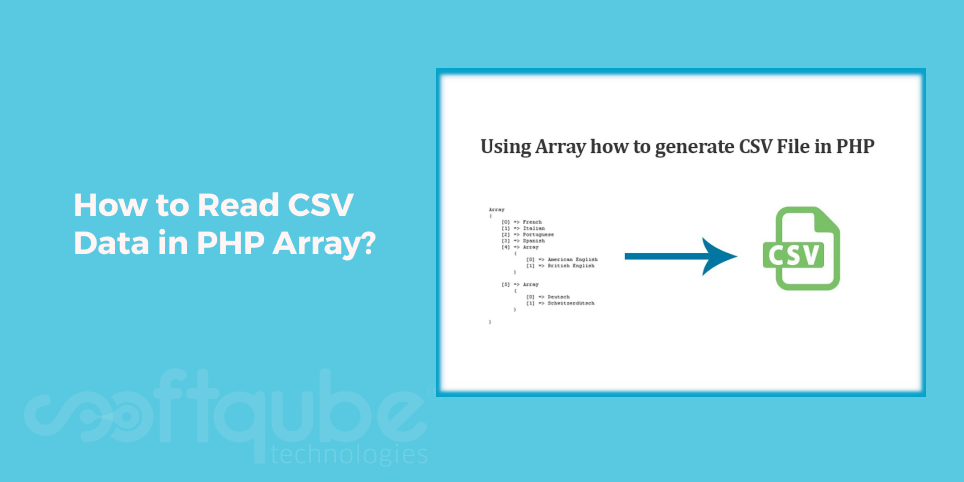
The CSV (“Comma Separated Values”) file format is an oldest form which is still used to exchange the data between different applications. It is been around for far longer personal computers. These CSV files are often used to import data from one application into another application like as MS Excel into database.
The import process is held with any programming language but here are few reasons to use PHP as one of the best for this purpose. Here, we will write a PHP Script which reads in a CSV file that transforms its contents into a multi dimensional array which is then to be inserted in WordPress database.
CSV Format:
The name CSV indicates the use of comma in order to separate data fields. CSV format can vary a little bit. Hence, one must not assume anything; just open the text editor and ensure that the format matches your expectations.
The field delimiter can be any character from tabs to the “I” symbol. Further, fields may be enclosed within single or double quotation marks and at times, the first record is a header that contains a list of field names.
One such sample file created by Excel’s Save As Command is given below. This includes header files and uses standard comma delimiter:
Store,Opening Hour Mon-Wed,OH Thurs,OH Fri,OH Sat,Sun
Aberdeen Beach,11:30-22:00,11:30-22:00,11:30-22:30,11:30-22:30,11:30-22:00
Basildon,12:00-22:30,12:00-22:30,12:00-23:00,12:00-23:00,12:00-22:30
Bath,12:00-22:30,12:00-22:30,12:00-23:00,12:00-23:00,11:30-22:30
Birmingham,11:30-23:00,11:30-23:00,11:30-23:00,11:30-23:00,11:30-23:00
Bluewater,12:00-22:30,12:00-22:30,12:00-23:00,12:00-23:00,12:00-22:30
Braehead,11:30-22:30,11:30-22:30,11:30-23:00,11:30-23:00,11:30-22:00
Braintree,12:00-22:30,12:00-22:30,12:00-23:00,12:00-23:00,12:00-22:00
...
Transforming File Entries into Associative Arrays:
PHP 5 is very well suited for reading in CSV files. With the file_get_contents() function, one can load the file contents in a string variable. Later, Explode () splits every file line into an array. Further, one needs to remove headers from the file using array_shift () function.
This is then fed into str_getcsv(). This is a specialized function that can be used for parsing CSV string into numerically indexed array. Here, one uses foreach loop in order to create a key for each field from the headers using array_combine ():
$csv = file_get_contents('./input_data.csv', FILE_USE_INCLUDE_PATH);
$lines = explode("\n", $csv);
//remove the first element from the array
$head = str_getcsv(array_shift($lines));
$data = array();
foreach ($lines as $line) {
$data[] = array_combine($head, str_getcsv($line));
}
This code will produce the following array construct:
array(72) {
[0]=>
array(6) {
["Store"]=>
string(14) "Aberdeen Beach"
["Opening Hour Mon-Wed"]=>
string(11) "11:30-22:00"
["OH Thurs"]=>
string(11) "11:30-22:00"
["OH Fri"]=>
string(11) "11:30-22:30"
["OH Sat"]=>
string(11) "11:30-22:30"
["Sun"]=>
string(11) "11:30-22:00"
}
[1]=>
array(6) {
["Store"]=>
string(8) "Basildon"
["Opening Hour Mon-Wed"]=>
string(11) "12:00-22:30"
["OH Thurs"]=>
string(11) "12:00-22:30"
["OH Fri"]=>
string(11) "12:00-23:00"
["OH Sat"]=>
string(11) "12:00-23:00"
["Sun"]=>
string(11) "12:00-22:30"
}
[2]=>
array(6) {
["Store"]=>
string(4) "Bath"
["Opening Hour Mon-Wed"]=>
string(11) "12:00-22:30"
["OH Thurs"]=>
string(11) "12:00-22:30"
["OH Fri"]=>
string(11) "12:00-23:00"
["OH Sat"]=>
string(11) "12:00-23:00"
["Sun"]=>
string(11) "11:30-22:30"
}
[3]=>
array(6) {
["Store"]=>
string(10) "Birmingham"
["Opening Hour Mon-Wed"]=>
string(11) "11:30-23:00"
["OH Thurs"]=>
string(11) "11:30-23:00"
["OH Fri"]=>
string(11) "11:30-23:00"
["OH Sat"]=>
string(11) "11:30-23:00"
["Sun"]=>
string(11) "11:30-23:00"
}
[4]=>
array(6) {
["Store"]=>
string(9) "Bluewater"
["Opening Hour Mon-Wed"]=>
string(11) "12:00-22:30"
["OH Thurs"]=>
string(11) "12:00-22:30"
["OH Fri"]=>
string(11) "12:00-23:00"
["OH Sat"]=>
string(11) "12:00-23:00"
["Sun"]=>
string(11) "12:00-22:30"
}
[5]=>
array(6) {
["Store"]=>
string(8) "Braehead"
["Opening Hour Mon-Wed"]=>
string(11) "11:30-22:30"
["OH Thurs"]=>
string(11) "11:30-22:30"
["OH Fri"]=>
string(11) "11:30-23:00"
["OH Sat"]=>
string(11) "11:30-23:00"
["Sun"]=>
string(11) "11:30-22:00"
}
//...
}
How to work with imported data?
When the CSV data is loaded into an array, then we are ready to load the data into system. Here, in this particular case; it involves fetching the post ID so that we can save location’s opening times as WordPress meta data.
Location name must be sanitized before inclusion in a query because it is not always that we can trust the external data. Each location array is passed to array_slice () function in order to separate the opening times from store name.
Inside foreach loop, data is formatted into HTML in order to display the label (array key), colon (: ), value on each line.
Take Away
Apart from specialized functions like as str_getcsv(), there’s another distinct advantage of using PHP Script to import CSV data is that it is very easy to set up as a cron job which runs at a given interval.
The file_get contents() function can accept a file URL so that it can retrieve every file directly from external partner’s server.
So, from now after reading this post; we are sure that you can easily import CSV data to PHP Array. Let us know your feedback about this and for more such news related to PHP, get in touch with Softqube Technologies providing PHP development in India at affordable costs.
Share on