.Net Reflection
January 13, 2014
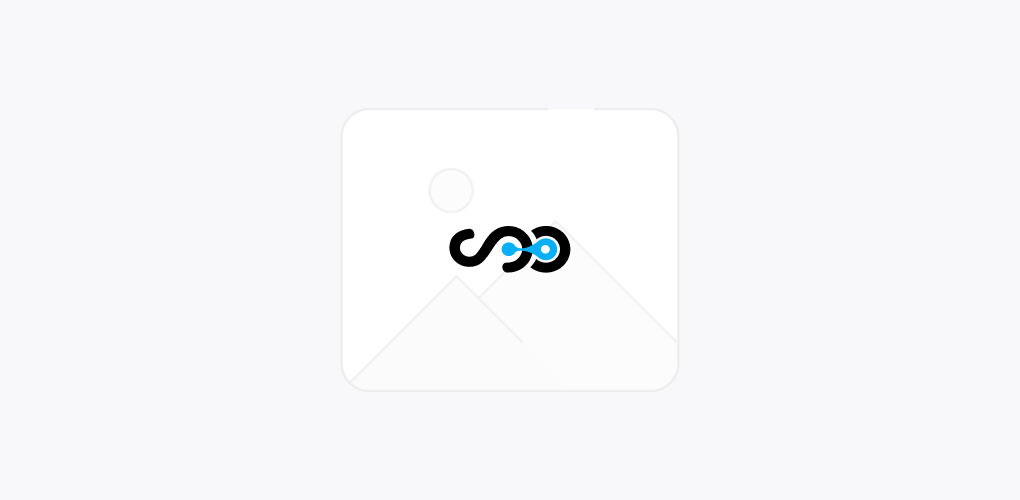
.Net provides best functionality of reflection to modify structure and behaviour of programming. .NET Framework’s Reflection API allows you to programmatically discover class information solely at run time.
In the computer science, .Net Reflection is the process in which the computer program can observe and modify its own structure and behaviour. It is mainly used to to load a .net assembly into memory programmatically. This is exactly how Reflection in C# works, and while you may not realize it at this point, being able to examine and change information about your application during runtime in C#.
Through the Reflection, you can get information on the methods, properties, fields, and events of an object, which you can see in a class viewer.
The System.Reflection namespace and the System.Type class play an important role in .NET Reflection. These allow you to reflect over many other aspects of a type. Below is the Demo1 and Demo2 for example to learn about reflection:
Demo1
using System;
using System.Reflection;
public class ReflactionDemo
{
public virtual int AdditionofNumber(int n1,int n2)
{
int result = n1 + n2;
return result;
}
}
class RefDemoMyRefClass
{
public static int Main()
{
Console.WriteLine (“MethodInfo”);
ReflactionDemo ReflactionDemoObj = new ReflactionDemo();
Type RefObj = ReflactionDemoObj.GetType();
MethodInfo DemoMethodInfo = RefObj.GetMethod(“AdditionofNumber”);
object[] RefParam = new object[] {5, 10};
Console.Write(“\nFirst method – ” + RefObj.FullName + ” returns ” + DemoMethodInfo.Invoke(ReflactionDemoObj, RefParam) + “\n”);
return 0;
}
}
get the type information
Type RefObj = Type.GetType(“ReflactionDemo”);
And RefObj will now have the required information about ReflactionDemo. Therefore we can now check if the class is an abstract class or a regular class
RefObj.IsClass or RefObj.IsAbstract
get the method’s information. And the method that we are interested in this case is AdditionofNumber
Demo2
Public class ReflactionDemo2
{
int answer;
public ReflactionDemo2()
{
answer = 0;
}
public int AdditionofNumber(intn1, intn2)
{
answer = n1 + n2;
return answer;
}
}
gets the System.Type object for the ReflactionDemo2 type.’
Type Reftype = typeof(ReflactionDemo2);
So we will now be able to create an instance of the Reftype object by passing the Reftype object to the Activator.CreateInstance(Reftype) method.
object obj = Activator.CreateInstance(Reftype);
Afterward, we can invoke the AdditionofNumber method of the ReflactionDemo2 class by first creating an array of objects for the arguments that we would be passing to the
AdditionofNumber(int, int) method.
object[] RefParam =newobject[] {5014, 6584};
Finally, we would invoke the AdditionofNumber(int, int) method by passing the method name AdditionofNumber to System.Type.InvokeMember() with the appropriate arguments.
int res = (int)Reftype.InvokeMember("AdditionofNumber", BindingFlags.InvokeMethod,null,obj, RefParam);
Here is the full Example of reflaction:
using System;
using System.Reflection;
namespace Reflection
{
class MyRefClass
{
public int AdditionofNumber(int n1, int n2)
{
int ans = n1 + n2;
return ans;
}
[STAThread]
static void Main(string[] args)
{
Type Reftype = typeof(MyRefClass);
object obj = Activator.CreateInstance(Reftype);
object[] RefParam = new object[] {5, 10};
int res = (int)Reftype.InvokeMember("AdditionofNumber", BindingFlags.InvokeMethod,null, obj, RefParam);
Console.Write("Result: {0} \n", res);
}
}
}
At Softqube, we provide high quality solutions with proven competency in web and .Net application development. For .Net reflection services, we serve you better with the required tools.
Share on